In Base - Part 2 we built out the base.njk
file adding the header and footer. Now that we have base file setup we can start adding pages, categories and posts.
Video Instructions
This video will walk you through this post and help explain each of the below steps.
Create the About Page
Create the about page by making an about
folder in the src
folder. Inside the about
folder create a index.html
file with the below HTML
to get started.
about > index.html
---
layout: 'base.njk'
tag: about-page
---
<section class="page">
<h1 class="container">About</h1>
<div class="container--bg about-page feature-box">
<p>Pellentesque lacinia ultrices purus ut scelerisque. Donec est lorem, commodo a ante vitae, feugiat cursus lectus. Nulla consequat et libero non sagittis.</p>
<h2>Pellentesque lacinia</h2>
<p>Pellentesque lacinia ultrices purus ut scelerisque. Donec est lorem, commodo a ante vitae, feugiat cursus lectus. Nulla consequat et libero non sagittis. Sed ut nisi et ligula tristique efficitur.</p>
</section>
We can add the about page title and meta description by adding an if-statement to the title-and-meta.njk
.
_includes > title-and-meta.njk
{%- if tag == 'home-page' -%}
<title>11ty Theme - by Design 2 SEO</title>
<meta name="description" content="This is the home page description.">
{%- elif tag == 'about-page' -%}
<title>11ty Theme - About</title>
<meta name="description" content="This is the about page description.">
{%- endif -%}
Create the Blog Category
Create the blog archives page by making a blog
folder in the src
folder. Inside the blog
folder create a index.html
file with the below front matter and Nunjucks to get started.
You can also use .njk
for the index file as there is really no HTML in this file.
blog > index.html
---
layout: 'base.njk'
tag: blog-page
eleventyExcludeFromCollections: true
---
{% include 'category.njk' %}
We will be including a category file that will sort all the categories for us.
Now we can create the category layout by making a category.njk
file in the _includes
folder with below code.
_includes > category.njk
<section class="container blog">
<div class="post--bg col-three">
{%- for post in collections.blog | reverse -%}
{%- include 'post-card.njk' -%}
{%- endfor -%}
</div>
</section>
Using a for-loop we loop through collections with the tags:
key that we will be setting to blog in the .json
file for the category. Now we need to create a post-card.njk
file in the _includes
folder with the below code.
_includes > post-card.njk
<article class="grid">
<div>
<a href="{{ post.url }}"><img src="{{ post.data.image }}" alt="" class="summary--image" width="1000" height="500"></a>
<p class="summary--title"><a href="{{ post.url }}">{{ post.data.title }}</a></p>
</div>
<span class="topic">
<a href="/blog/{{ post.data.categorySlug }}" class="btn btn--primary">{{ post.data.categoryName }}</a>
</span>
</article>
This will create the post summary or in this case a card with the image, title and category. This data gets called from the post front matter and the folders .json
file.
Sub Category and Post
Create the sub category by making a topic-one
folder in the blog
folder. Inside the topic-one
folder create a category archives page by making a index.html
file.
You can also use .njk
for the index file as there is really no HTML in this file.
blog > topic-one > index.html
---
layout: 'base.njk'
tag: topic-one-page
eleventyExcludeFromCollections: true
---
{% include 'category.njk' %}
To get the corresponding posts for this category pages use the if
else
statements and elif
statement as you add more categories in the category.njk
file.
_includes > category.njk
<section class="container blog">
<div class="post--bg col-three">
{%- if tag == 'topic-one-page' -%}
{%- for post in collections.topicOnePosts | reverse -%}
{%- include 'post-card.njk' -%}
{%- endfor -%}
{%- else -%}
{%- for post in collections.blog | reverse -%}
{%- include 'post-card.njk' -%}
{%- endfor -%}
{% endif %}
</div>
</section>
Create a post by making a first-post.html
file with the below front matter and HTML to get started.
blog > topic-one > first-post.html
---
title: First Post
date: 2022-04-24
image: /assets/images/design2seo-11ty-theme.jpg
imageAlt: I'm baby fashion axe swag actually, retro man
description: I'm baby fashion axe swag actually, retro man bun migas photo booth palo santo. Unicorn chillwave pork belly put a bird on it selvage gastropub celiac migas gochujang pour-over locavore keytar man braid sustainable shabby chic.
---
<figure><img src="/assets/images/design2seo-11ty-theme.jpg" alt="" width="1000" height="500"><figcaption class="med">11ty Theme by Design 2 SEO</figcaption></figure>
<p>YOLO pop-up synth fixie slow-carb fingerstache before they sold out twee readymade church-key DIY meh umami crucifix offal. PBR&B craft beer photo booth lyft gastropub 90's.</p>
<h2>This is a H2 Heading</h2>
<div class="one-third pad-pad">
<img src="/assets/images/design2seo-11ty-theme.jpg" alt=""><div>
<p class="no-top no-bottom">I'm baby fashion axe swag actually, retro man bun migas photo booth palo santo. Unicorn chillwave pork belly put a bird on it selvage gastropub.</p>
</div>
</div>
<p>YOLO pop-up synth fixie slow-carb fingerstache before they sold out twee readymade church-key DIY meh umami crucifix offal. PBR&B craft beer photo booth lyft gastropub 90's.</p>
<p>Chicharrones schlitz austin tote bag, flannel flexitarian hell of tilde etsy polaroid taxidermy pour-over humblebrag squid. Listicle 90's hot chicken, messenger bag vegan activated charcoal banh mi.</p>
Create the sub category data by making a topic-one.json
file in the topic-one
folder. 11ty will use this file to add the data to each of the posts, so we don't have to manually add it to each one in the front matter. This is where we will put the repeating data like layout, author, collections, page, slug and category.
blog > topic-one > topic-one.json
{
"layout": "post.njk",
"author": "admin",
"tags": ["topicOnePosts", "blog"],
"tag": ["topic-one"],
"categorySlug": "topic-one/",
"categoryName": "Topic One"
}
Now anytime we call the blog with collections we will get all to posts with the tags:
key labeled blog. I also added topic-one
to the tags:
key so we can grab only the posts from the Topic One category.
Post Layout
Now we need to create our post layout that will give us the title, author, date and div structure for the posts.
Make a post.njk
file in the _includes
folder with below code.
_includes > post.njk
---
layout: "base.njk"
---
<section class="container post">
<h1 class="article--title">{{ title }}</h1>
<div class="author">By: <a href="/about/" title="Posts by admin" rel="author">{{ author }}</a> on {{ date | postDate }}</div>
<article class="post--bg">
{{ content | safe }}
</article>
</section>
Add the post image by creating a assets
folder and a images
folder inside of that. They will go in the src
folder. The image for the card will come from the images:
key in the post front matter as well as the image alt:
.
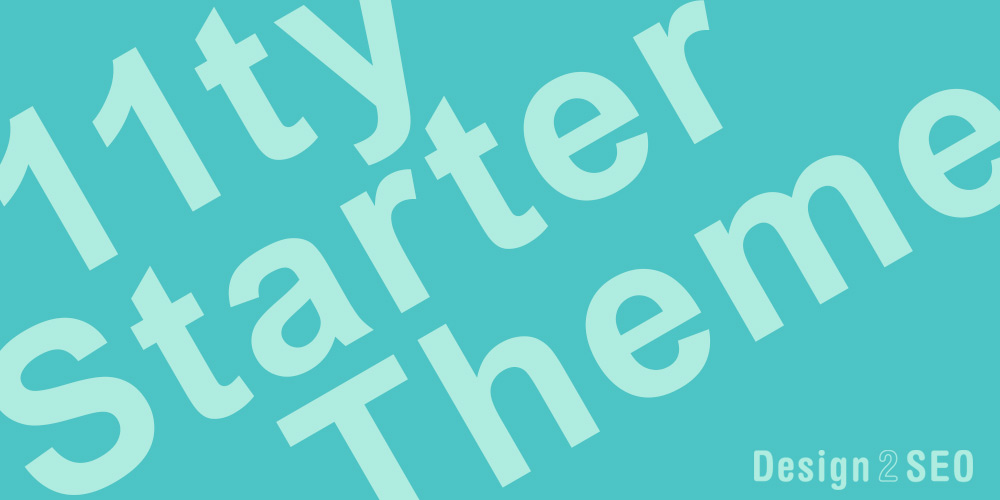
Here is the themes 1000px by 500px place holder image to use in the images folder.
Finally we need to add a couple things to the .eleventy
file. The date filter to formate the post date for us and a pass through function for the images folder. So your .eleventy
file should now look like this.
.eleventy.js
const { DateTime } = require("luxon");
module.exports = function(eleventyConfig) {
//Pass through files
eleventyConfig.addPassthroughCopy('./src/style.css');
eleventyConfig.addPassthroughCopy('./src/assets');
//Date Clean up
eleventyConfig.addFilter("postDate", (dateObj) => {
return DateTime.fromJSDate(dateObj).toLocaleString(DateTime.DATE_MED);
});
return {
dir: {
input: "src"
}
}
};
We include the built in luxon
tool at the top of the file and then we formate the date in the eleventyConfig
function.
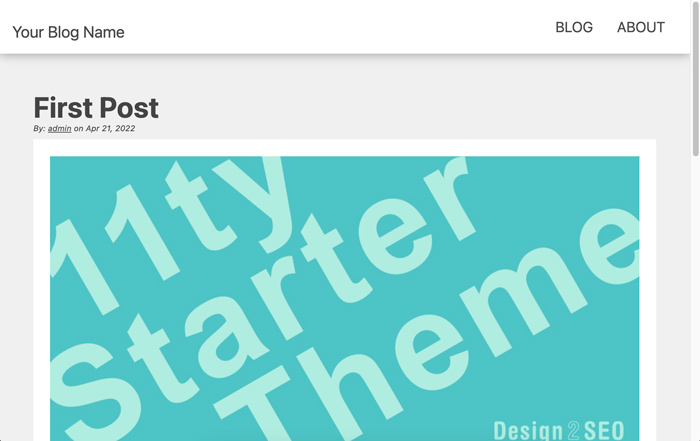
Now we can go to the terminal for the project folder and type:npm start
and go to http://localhost:8080 and we should see our finished blog template!
Make a habit of deleting the _site
build fold folder anytime you are changing file names. Then restart the terminal by pressing "control + C" and restart it.
Conclusion
This is the base for building a blog with 11ty. I will continue to make posts on adding features like active links, breadcrumbs, summaries and more. There is no limit to what you can do with 11ty!
If you have any question leave me a message in the comment of the Youtube video and I will return your comment.
This process may seem like allot to build, but in the end, once the setup is done, you will come out way ahead of all the other frameworks and platforms. So if you are looking for a light weight easy to manage site that can be hosted for free on Netlify - I recommend Eleventy.
Follow along on GitHub - Part 3.